Main Content
Avoider (Part 2)
A copy of the full source code for today's tutorial is available
Welcome to part two of this Foundation Flash free tutorial, about the making of an Avoider type game, the second in a series of Foundation Flash classic game remakes. The game we shall be making is shown below. You are the black circle, try and get the blue squares but avoider the white, 'enemy' circles.
Well, that's the slight rewrite of what I said last week out of the way (oh, the beauties of copy and paste!). THe week we are going to be actually doing something. Sharp intake of breath. From last week, you should have four Movie Clips for the walls, with which we shall be interacting either this week or next. For now though, we will turn our attention to the player.
Go to your ActionScript, and inside the constructor - the Avoider() function - call a new function, which we are about to create, called drawPlayer(). In case you don't know how to do that (and you really should by now), it's like this:
drawPlayer();
There. Now, of course, we have to make a function called drawPlayer. We does this in the standard way, which is all explained in another tutorial:
public function drawPlayer() { }
Now we need to draw the player within that function. For this we are going to be using that Movie Clip we made last week called 'player', just adding some graphic details. For an explanation of them, please see earlier tutorials (perhap this one?
). The full code for this is shown below.player.graphics.beginFill(0x000000); player.graphics.drawCircle(0, 0, 10); player.x = 200; player.y = 200; player.graphics.endFill(); addChild(player);
I think, perhaps, the only important thing to note from that is that the registration point of the circle (its (0,0)) is in the middle of it, not in the top left hand corner. This makes the game that little bit better when you move using the mouse in this way. If you're wondering why, look at the three parameters of the drawCircle function: x of the middle of the circle, y of the middle of the circle and radius. Compiling your movie at this point will show you (oh joy of joys!) that we now have a black circle on the stage. A black circle that doesn't move. Well, now it shall.
Go back to the constructor function and, underneath the drawPlayer function, add in this line:
addEventListener(Event.ENTER_FRAME, mouseMovement);
We first mentioned Event listeners way back when so you should know about them already. As you can see, every frame, the mouseMovement frame will be called. Wait a minute! We don't have a mouseMovement function. Oh yes we do (as of now):
public function mouseMovement(event:Event):void { mouseDiffY = mouseY - player.y; mouseDiffX = mouseX - player.x; }
We simply calculate the current mouseDiffs, as noted last week, but we're still not actually doing anything. THere are four more blocks of code to add UNDER those two lines, I will talk you through one and you can copy and paste the other three. Firstly though, here is a helpful diagram showing the various mouseDiffs:
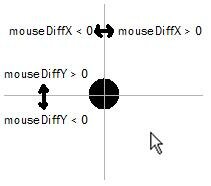
And here is that one, which handles all negative Y differences:
if (mouseDiffY < 0) { if (!player.hitTestObject(walltop)) { player.y += mouseDiffY / 6; } }
We also have a tutorial about the hitTestObject function as well. Basically what we say here is that "If we are below the player, and not touching the top wall, move closer to them on the y-axis." n.b. Not upwards, towards. And, finally for today, the other three:
if (mouseDiffY > 0) { if (!player.hitTestObject(wallbottom)) { player.y += mouseDiffY / 6; } } if (mouseDiffX < 0) { if (!player.hitTestObject(wallleft)) { player.x += mouseDiffX / 6; } } if (mouseDiffX > 0) { if (!player.hitTestObject(wallright)) { player.x += mouseDiffX / 6; } }
If you now compile your game (CTRL/Command + Enter) you should see that the player moves towards the mouse, which is obviously an integral part of the game. Next week: adding in the targets and scoring. Please join me for that free Foundation Flash tutorial.
Harry.